Staging
v0.5.1
v0.5.1
https://github.com/python/cpython
Revision a2b7f40513ba5d75a2063c3fabe47377cd8c0416 authored by Guido van Rossum on 04 January 1993, 09:09:59 UTC, committed by Guido van Rossum on 04 January 1993, 09:09:59 UTC
* posixmodule.c: move extern function declarations to top * listobject.c: cmp() arguments must be void* if __STDC__ * Makefile, allobjects.h, panelmodule.c, modsupport.c: get rid of strdup() -- it is a portability risk * Makefile: enclosed ranlib command in parentheses for Sequent Make which aborts if the command is not found even if '-' is present * timemodule.c: time() returns a floating point number, in microsecond precision if BSD_TIME is defined.
1 parent 349f2b5
Tip revision: a2b7f40513ba5d75a2063c3fabe47377cd8c0416 authored by Guido van Rossum on 04 January 1993, 09:09:59 UTC
* Configure.py: use #!/usr/local/bin/python
* Configure.py: use #!/usr/local/bin/python
Tip revision: a2b7f40
moduleobject.c
/***********************************************************
Copyright 1991, 1992 by Stichting Mathematisch Centrum, Amsterdam, The
Netherlands.
All Rights Reserved
Permission to use, copy, modify, and distribute this software and its
documentation for any purpose and without fee is hereby granted,
provided that the above copyright notice appear in all copies and that
both that copyright notice and this permission notice appear in
supporting documentation, and that the names of Stichting Mathematisch
Centrum or CWI not be used in advertising or publicity pertaining to
distribution of the software without specific, written prior permission.
STICHTING MATHEMATISCH CENTRUM DISCLAIMS ALL WARRANTIES WITH REGARD TO
THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
FITNESS, IN NO EVENT SHALL STICHTING MATHEMATISCH CENTRUM BE LIABLE
FOR ANY SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT
OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
******************************************************************/
/* Module object implementation */
#include "allobjects.h"
typedef struct {
OB_HEAD
object *md_name;
object *md_dict;
} moduleobject;
object *
newmoduleobject(name)
char *name;
{
moduleobject *m = NEWOBJ(moduleobject, &Moduletype);
if (m == NULL)
return NULL;
m->md_name = newstringobject(name);
m->md_dict = newdictobject();
if (m->md_name == NULL || m->md_dict == NULL) {
DECREF(m);
return NULL;
}
return (object *)m;
}
object *
getmoduledict(m)
object *m;
{
if (!is_moduleobject(m)) {
err_badcall();
return NULL;
}
return ((moduleobject *)m) -> md_dict;
}
char *
getmodulename(m)
object *m;
{
if (!is_moduleobject(m)) {
err_badarg();
return NULL;
}
return getstringvalue(((moduleobject *)m) -> md_name);
}
/* Methods */
static void
module_dealloc(m)
moduleobject *m;
{
if (m->md_name != NULL)
DECREF(m->md_name);
if (m->md_dict != NULL)
DECREF(m->md_dict);
free((char *)m);
}
static object *
module_repr(m)
moduleobject *m;
{
char buf[100];
sprintf(buf, "<module '%.80s'>", getstringvalue(m->md_name));
return newstringobject(buf);
}
static object *
module_getattr(m, name)
moduleobject *m;
char *name;
{
object *res;
if (strcmp(name, "__dict__") == 0) {
INCREF(m->md_dict);
return m->md_dict;
}
if (strcmp(name, "__name__") == 0) {
INCREF(m->md_name);
return m->md_name;
}
res = dictlookup(m->md_dict, name);
if (res == NULL)
err_setstr(AttributeError, name);
else
INCREF(res);
return res;
}
static int
module_setattr(m, name, v)
moduleobject *m;
char *name;
object *v;
{
if (strcmp(name, "__dict__") == 0 || strcmp(name, "__name__") == 0) {
err_setstr(TypeError, "read-only special attribute");
return -1;
}
if (v == NULL) {
int rv = dictremove(m->md_dict, name);
if (rv < 0)
err_setstr(AttributeError,
"delete non-existing module attribute");
return rv;
}
else
return dictinsert(m->md_dict, name, v);
}
typeobject Moduletype = {
OB_HEAD_INIT(&Typetype)
0, /*ob_size*/
"module", /*tp_name*/
sizeof(moduleobject), /*tp_size*/
0, /*tp_itemsize*/
module_dealloc, /*tp_dealloc*/
0, /*tp_print*/
module_getattr, /*tp_getattr*/
module_setattr, /*tp_setattr*/
0, /*tp_compare*/
module_repr, /*tp_repr*/
};
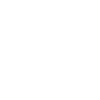
Computing file changes ...