Staging
v0.5.2
v0.5.2
https://github.com/git/git
Revision 6490a3383f1d0d96c122069e510ef1af1d019fbb authored by Junio C Hamano on 02 August 2007, 22:10:56 UTC, committed by Junio C Hamano on 03 August 2007, 00:26:26 UTC
In set_work_tree(), variable rel needs to be reinitialized to NULL on every call (it should not be static). Make sure the incoming dir variable is not too long before copying to the temporary buffer, and make sure chdir to the resulting directory succeeds. This was spotted and fixed by Alex and Johannes in a handful patch exchanges. Here is the final version. Signed-off-by: Junio C Hamano <gitster@pobox.com> Acked-by: Johannes Schindelin <Johannes.Schindelin@gmx.de>
1 parent 29093c2
Tip revision: 6490a3383f1d0d96c122069e510ef1af1d019fbb authored by Junio C Hamano on 02 August 2007, 22:10:56 UTC
Fix work-tree related breakages
Fix work-tree related breakages
Tip revision: 6490a33
write_or_die.c
#include "cache.h"
/*
* Some cases use stdio, but want to flush after the write
* to get error handling (and to get better interactive
* behaviour - not buffering excessively).
*
* Of course, if the flush happened within the write itself,
* we've already lost the error code, and cannot report it any
* more. So we just ignore that case instead (and hope we get
* the right error code on the flush).
*
* If the file handle is stdout, and stdout is a file, then skip the
* flush entirely since it's not needed.
*/
void maybe_flush_or_die(FILE *f, const char *desc)
{
static int skip_stdout_flush = -1;
struct stat st;
char *cp;
if (f == stdout) {
if (skip_stdout_flush < 0) {
cp = getenv("GIT_FLUSH");
if (cp)
skip_stdout_flush = (atoi(cp) == 0);
else if ((fstat(fileno(stdout), &st) == 0) &&
S_ISREG(st.st_mode))
skip_stdout_flush = 1;
else
skip_stdout_flush = 0;
}
if (skip_stdout_flush && !ferror(f))
return;
}
if (fflush(f)) {
if (errno == EPIPE)
exit(0);
die("write failure on %s: %s", desc, strerror(errno));
}
}
int read_in_full(int fd, void *buf, size_t count)
{
char *p = buf;
ssize_t total = 0;
while (count > 0) {
ssize_t loaded = xread(fd, p, count);
if (loaded <= 0)
return total ? total : loaded;
count -= loaded;
p += loaded;
total += loaded;
}
return total;
}
int write_in_full(int fd, const void *buf, size_t count)
{
const char *p = buf;
ssize_t total = 0;
while (count > 0) {
ssize_t written = xwrite(fd, p, count);
if (written < 0)
return -1;
if (!written) {
errno = ENOSPC;
return -1;
}
count -= written;
p += written;
total += written;
}
return total;
}
void write_or_die(int fd, const void *buf, size_t count)
{
if (write_in_full(fd, buf, count) < 0) {
if (errno == EPIPE)
exit(0);
die("write error (%s)", strerror(errno));
}
}
int write_or_whine_pipe(int fd, const void *buf, size_t count, const char *msg)
{
if (write_in_full(fd, buf, count) < 0) {
if (errno == EPIPE)
exit(0);
fprintf(stderr, "%s: write error (%s)\n",
msg, strerror(errno));
return 0;
}
return 1;
}
int write_or_whine(int fd, const void *buf, size_t count, const char *msg)
{
if (write_in_full(fd, buf, count) < 0) {
fprintf(stderr, "%s: write error (%s)\n",
msg, strerror(errno));
return 0;
}
return 1;
}
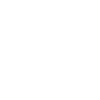
Computing file changes ...