Staging
v0.5.1
v0.5.1
https://github.com/python/cpython
- HEAD
- refs/heads/2.7
- refs/heads/3.6
- refs/heads/3.7
- refs/heads/3.8
- refs/heads/3.9
- refs/heads/Mariatta-patch-1
- refs/heads/Mariatta-patch-2
- refs/heads/buildbot-custom
- refs/heads/descr-howto-feedback
- refs/heads/master
- refs/heads/revert-23107-revert-13893-fix-issue-37193
- refs/tags/v0.9.8
- refs/tags/v0.9.9
- refs/tags/v1.0.1
- refs/tags/v1.0.2
- refs/tags/v1.1
- refs/tags/v1.1.1
- refs/tags/v1.2
- refs/tags/v1.2b1
- refs/tags/v1.2b2
- refs/tags/v1.2b3
- refs/tags/v1.2b4
- refs/tags/v1.3
- refs/tags/v1.3b1
- refs/tags/v1.4
- refs/tags/v1.4b1
- refs/tags/v1.4b2
- refs/tags/v1.4b3
- refs/tags/v1.5
- refs/tags/v1.5.1
- refs/tags/v1.5.2
- refs/tags/v1.5.2a1
- refs/tags/v1.5.2a2
- refs/tags/v1.5.2b1
- refs/tags/v1.5.2b2
- refs/tags/v1.5.2c1
- refs/tags/v1.5a1
- refs/tags/v1.5a2
- refs/tags/v1.5a3
- refs/tags/v1.5a4
- refs/tags/v1.5b1
- refs/tags/v1.5b2
- refs/tags/v1.6a1
- refs/tags/v1.6a2
- refs/tags/v2.0
- refs/tags/v2.0.1
- refs/tags/v2.0.1c1
- refs/tags/v2.0b1
- refs/tags/v2.0b2
- refs/tags/v2.0c1
- refs/tags/v2.1
- refs/tags/v2.1.1
- refs/tags/v2.1.1c1
- refs/tags/v2.1.2
- refs/tags/v2.1.2c1
- refs/tags/v2.1.3
- refs/tags/v2.1a1
- refs/tags/v2.1a2
- refs/tags/v2.1b1
- refs/tags/v2.1b2
- refs/tags/v2.1c1
- refs/tags/v2.1c2
- refs/tags/v2.2
- refs/tags/v2.2.1
- refs/tags/v2.2.1c1
- refs/tags/v2.2.1c2
- refs/tags/v2.2.2
- refs/tags/v2.2.2b1
- refs/tags/v2.2.3
- refs/tags/v2.2.3c1
- refs/tags/v2.2a3
- refs/tags/v2.3.1
- refs/tags/v2.3.2
- refs/tags/v2.3.2c1
- refs/tags/v2.3.3
- refs/tags/v2.3.3c1
- refs/tags/v2.3.4
- refs/tags/v2.3.4c1
- refs/tags/v2.3.5
- refs/tags/v2.3.5c1
- refs/tags/v2.3.6
- refs/tags/v2.3.6c1
- refs/tags/v2.3.7
- refs/tags/v2.3.7c1
- refs/tags/v2.3c1
- refs/tags/v2.3c2
- refs/tags/v2.4
- refs/tags/v2.4.1
- refs/tags/v2.4.1c1
- refs/tags/v2.4.1c2
- refs/tags/v2.4.2
- refs/tags/v2.4.2c1
- refs/tags/v2.4.3
- refs/tags/v2.4.3c1
- refs/tags/v2.4.4
- refs/tags/v2.4.4c1
- refs/tags/v2.4.5
- refs/tags/v2.4.5c1
- refs/tags/v2.4.6
- refs/tags/v2.4.6c1
- refs/tags/v2.4a1
- refs/tags/v2.4a2
- refs/tags/v2.4a3
- refs/tags/v2.4b1
- refs/tags/v2.4b2
- refs/tags/v2.4c1
- refs/tags/v2.5
- refs/tags/v2.5.1
- refs/tags/v2.5.1c1
- refs/tags/v2.5.2
- refs/tags/v2.5.2c1
- refs/tags/v2.5.3
- refs/tags/v2.5.3c1
- refs/tags/v2.5.4
- refs/tags/v2.5.5
- refs/tags/v2.5.5c1
- refs/tags/v2.5.5c2
- refs/tags/v2.5.6
- refs/tags/v2.5.6c1
- refs/tags/v2.5a0
- refs/tags/v2.5a1
- refs/tags/v2.5a2
- refs/tags/v2.5b1
- refs/tags/v2.5b2
- refs/tags/v2.5b3
- refs/tags/v2.5c1
- refs/tags/v2.5c2
- refs/tags/v2.6
- refs/tags/v2.6.1
- refs/tags/v2.6.2
- refs/tags/v2.6.2c1
- refs/tags/v2.6.3
- refs/tags/v2.6.3rc1
- refs/tags/v2.6.4
- refs/tags/v2.6.4rc1
- refs/tags/v2.6.4rc2
- refs/tags/v2.6.5
- refs/tags/v2.6.5rc1
- refs/tags/v2.6.5rc2
- refs/tags/v2.6.6
- refs/tags/v2.6.6rc1
- refs/tags/v2.6.6rc2
- refs/tags/v2.6.7
- refs/tags/v2.6.8
- refs/tags/v2.6.8rc1
- refs/tags/v2.6.8rc2
- refs/tags/v2.6.9
- refs/tags/v2.6.9rc1
- refs/tags/v2.6a1
- refs/tags/v2.6a2
- refs/tags/v2.6a3
- refs/tags/v2.6b1
- refs/tags/v2.6b2
- refs/tags/v2.6b3
- refs/tags/v2.6rc1
- refs/tags/v2.6rc2
- refs/tags/v2.7
- refs/tags/v2.7.1
- refs/tags/v2.7.10
- refs/tags/v2.7.10rc1
- refs/tags/v2.7.11
- refs/tags/v2.7.11rc1
- refs/tags/v2.7.12
- refs/tags/v2.7.12rc1
- refs/tags/v2.7.13
- refs/tags/v2.7.13rc1
- refs/tags/v2.7.1rc1
- refs/tags/v2.7.2
- refs/tags/v2.7.2rc1
- refs/tags/v2.7.3
- refs/tags/v2.7.3rc1
- refs/tags/v2.7.3rc2
- refs/tags/v2.7.4
- refs/tags/v2.7.4rc1
- refs/tags/v2.7.5
- refs/tags/v2.7.6
- refs/tags/v2.7.6rc1
- refs/tags/v2.7.7
- refs/tags/v2.7.7rc1
- refs/tags/v2.7.8
- refs/tags/v2.7.9
- refs/tags/v2.7.9rc1
- refs/tags/v2.7a1
- refs/tags/v2.7a2
- refs/tags/v2.7a3
- refs/tags/v2.7a4
- refs/tags/v2.7b1
- refs/tags/v2.7b2
- refs/tags/v2.7rc1
- refs/tags/v2.7rc2
- refs/tags/v3.0
- refs/tags/v3.0.1
- refs/tags/v3.0a1
- refs/tags/v3.0a2
- refs/tags/v3.0a3
- refs/tags/v3.0a4
- refs/tags/v3.0a5
- refs/tags/v3.0b1
- refs/tags/v3.0b2
- refs/tags/v3.0b3
- refs/tags/v3.0rc1
- refs/tags/v3.0rc2
- refs/tags/v3.0rc3
- refs/tags/v3.1
- refs/tags/v3.1.1
- refs/tags/v3.1.1rc1
- refs/tags/v3.1.2
- refs/tags/v3.1.2rc1
- refs/tags/v3.1.3
- refs/tags/v3.1.3rc1
- refs/tags/v3.1.4
- refs/tags/v3.1.4rc1
- refs/tags/v3.1.5
- refs/tags/v3.1.5rc1
- refs/tags/v3.1.5rc2
- refs/tags/v3.1a1
- refs/tags/v3.1a2
- refs/tags/v3.1b1
- refs/tags/v3.1rc1
- refs/tags/v3.1rc2
- refs/tags/v3.2
- refs/tags/v3.2.1
- refs/tags/v3.2.1b1
- refs/tags/v3.2.1rc1
- refs/tags/v3.2.1rc2
- refs/tags/v3.2.2
- refs/tags/v3.2.2rc1
- refs/tags/v3.2.3
- refs/tags/v3.2.3rc1
- refs/tags/v3.2.3rc2
- refs/tags/v3.2.4
- refs/tags/v3.2.4rc1
- refs/tags/v3.2.5
- refs/tags/v3.2.6
- refs/tags/v3.2.6rc1
- refs/tags/v3.2a1
- refs/tags/v3.2a2
- refs/tags/v3.2a3
- refs/tags/v3.2a4
- refs/tags/v3.2b1
- refs/tags/v3.2b2
- refs/tags/v3.2rc1
- refs/tags/v3.2rc2
- refs/tags/v3.2rc3
- refs/tags/v3.3.0
- refs/tags/v3.3.0a1
- refs/tags/v3.3.0a2
- refs/tags/v3.3.0a3
- refs/tags/v3.3.0a4
- refs/tags/v3.3.0b1
- refs/tags/v3.3.0b2
- refs/tags/v3.3.0rc1
- refs/tags/v3.3.0rc2
- refs/tags/v3.3.0rc3
- refs/tags/v3.3.1
- refs/tags/v3.3.1rc1
- refs/tags/v3.3.2
- refs/tags/v3.3.3
- refs/tags/v3.3.3rc1
- refs/tags/v3.3.3rc2
- refs/tags/v3.3.4
- refs/tags/v3.3.4rc1
- refs/tags/v3.3.5
- refs/tags/v3.3.5rc1
- refs/tags/v3.3.5rc2
- refs/tags/v3.3.6
- refs/tags/v3.3.6rc1
- refs/tags/v3.4.0
- refs/tags/v3.4.0a1
- refs/tags/v3.4.0a2
- refs/tags/v3.4.0a3
- refs/tags/v3.4.0a4
- refs/tags/v3.4.0b1
- refs/tags/v3.4.0b2
- refs/tags/v3.4.0b3
- refs/tags/v3.4.0rc1
- refs/tags/v3.4.0rc2
- refs/tags/v3.4.0rc3
- refs/tags/v3.4.1
- refs/tags/v3.4.1rc1
- refs/tags/v3.4.2
- refs/tags/v3.4.2rc1
- refs/tags/v3.4.3
- refs/tags/v3.4.3rc1
- refs/tags/v3.4.4
- refs/tags/v3.4.4rc1
- refs/tags/v3.4.5
- refs/tags/v3.4.5rc1
- refs/tags/v3.4.6
- refs/tags/v3.4.6rc1
- refs/tags/v3.5.0
- refs/tags/v3.5.0a1
- refs/tags/v3.5.0a2
- refs/tags/v3.5.0a3
- refs/tags/v3.5.0a4
- refs/tags/v3.5.0b1
- refs/tags/v3.5.0b2
- refs/tags/v3.5.0b3
- refs/tags/v3.5.0b4
- refs/tags/v3.5.0rc1
- refs/tags/v3.5.0rc2
- refs/tags/v3.5.0rc3
- refs/tags/v3.5.0rc4
- refs/tags/v3.5.1
- refs/tags/v3.5.1rc1
- refs/tags/v3.5.2
- refs/tags/v3.5.2rc1
- refs/tags/v3.5.3
- refs/tags/v3.5.3rc1
- refs/tags/v3.6.0
- refs/tags/v3.6.0a1
- refs/tags/v3.6.0a2
- refs/tags/v3.6.0a3
- refs/tags/v3.6.0a4
- refs/tags/v3.6.0b1
- refs/tags/v3.6.0b2
- refs/tags/v3.6.0b3
- refs/tags/v3.6.0b4
- refs/tags/v3.6.0rc1
- refs/tags/v3.6.0rc2
- refs/tags/v3.9.0a1
- refs/tags/v3.9.0a6
- v3.9.1rc1
- v3.9.1
- v3.9.0rc2
- v3.9.0rc1
- v3.9.0b5
- v3.9.0b4
- v3.9.0b3
- v3.9.0b2
- v3.9.0b1
- v3.9.0a5
- v3.9.0a4
- v3.9.0a3
- v3.9.0a2
- v3.9.0
- v3.8.7rc1
- v3.8.6rc1
- v3.8.6
- v3.8.5
- v3.8.4rc1
- v3.8.4
- v3.8.3rc1
- v3.8.3
- v3.8.2rc2
- v3.8.2rc1
- v3.8.2
- v3.8.1rc1
- v3.8.1
- v3.8.0rc1
- v3.8.0b4
- v3.8.0b3
- v3.8.0b2
- v3.8.0b1
- v3.8.0a4
- v3.8.0a3
- v3.8.0a2
- v3.8.0a1
- v3.8.0
- v3.7.9
- v3.7.8rc1
- v3.7.8
- v3.7.7rc1
- v3.7.7
- v3.7.6rc1
- v3.7.6
- v3.7.5rc1
- v3.7.5
- v3.7.4rc2
- v3.7.4rc1
- v3.7.4
- v3.7.3rc1
- v3.7.3
- v3.7.2rc1
- v3.7.2
- v3.7.1rc2
- v3.7.1rc1
- v3.7.1
- v3.7.0rc1
- v3.7.0b5
- v3.7.0b4
- v3.7.0b3
- v3.7.0b2
- v3.7.0b1
- v3.7.0a4
- v3.7.0a3
- v3.7.0a2
- v3.7.0a1
- v3.7.0
- v3.6.9rc1
- v3.6.9
- v3.6.8rc1
- v3.6.8
- v3.6.7rc2
- v3.6.7rc1
- v3.6.7
- v3.6.6rc1
- v3.6.6
- v3.6.5rc1
- v3.6.5
- v3.6.4rc1
- v3.6.4
- v3.6.3rc1
- v3.6.3
- v3.6.2rc2
- v3.6.2rc1
- v3.6.2
- v3.6.1rc1
- v3.6.12
- v3.6.11rc1
- v3.6.11
- v3.6.10rc1
- v3.6.10
- v3.6.1
- v3.5.9
- v3.5.8rc2
- v3.5.8rc1
- v3.5.8
- v3.5.7rc1
- v3.5.7
- v3.5.6rc1
- v3.5.6
- v3.5.5rc1
- v3.5.5
- v3.5.4rc1
- v3.5.4
- v3.5.10rc1
- v3.5.10
- v3.4.9rc1
- v3.4.9
- v3.4.8rc1
- v3.4.8
- v3.4.7rc1
- v3.4.7
- v3.4.10rc1
- v3.4.10
- v3.3.7rc1
- v3.3.7
- v3.10.0a3
- v3.10.0a2
- v3.10.0a1
- v2.7.18rc1
- v2.7.18
- v2.7.17rc1
- v2.7.17
- v2.7.16rc1
- v2.7.16
- v2.7.15rc1
- v2.7.15
- v2.7.14rc1
- v2.7.14
- legacy-trunk
- 3.5
- 3.4
- 3.3
- 3.2
- 3.1
- 3.0
- 2.6
- 2.5
- 2.4
- 2.3
- 2.2
- 2.1
- 2.0
Raw File
Take a new snapshot of a software origin
If the archived software origin currently browsed is not synchronized with its upstream version (for instance when new commits have been issued), you can explicitly request Software Heritage to take a new snapshot of it.
Use the form below to proceed. Once a request has been submitted and accepted, it will be processed as soon as possible. You can then check its processing state by visiting this dedicated page.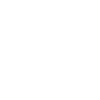
Processing "take a new snapshot" request ...
Permalinks
To reference or cite the objects present in the Software Heritage archive, permalinks based on SoftWare Hash IDentifiers (SWHIDs) must be used.
Select below a type of object currently browsed in order to display its associated SWHID and permalink.
Tip revision: 07c9d8547c39809282884bf0189d977b5e4fec4f authored by Ned Deily on 09 January 2018, 03:05:14 UTC
Bump to 3.7.0a4
Bump to 3.7.0a4
Tip revision: 07c9d85
_xxtestfuzz.c
#define PY_SSIZE_T_CLEAN
#include <Python.h>
#include <stdlib.h>
#include <inttypes.h>
int LLVMFuzzerTestOneInput(const uint8_t *data, size_t size);
static PyObject* _fuzz_run(PyObject* self, PyObject* args) {
const char* buf;
Py_ssize_t size;
if (!PyArg_ParseTuple(args, "s#", &buf, &size)) {
return NULL;
}
int rv = LLVMFuzzerTestOneInput((const uint8_t*)buf, size);
if (PyErr_Occurred()) {
return NULL;
}
if (rv != 0) {
// Nonzero return codes are reserved for future use.
PyErr_Format(
PyExc_RuntimeError, "Nonzero return code from fuzzer: %d", rv);
return NULL;
}
Py_RETURN_NONE;
}
static PyMethodDef module_methods[] = {
{"run", (PyCFunction)_fuzz_run, METH_VARARGS, ""},
{NULL},
};
static struct PyModuleDef _fuzzmodule = {
PyModuleDef_HEAD_INIT,
"_fuzz",
NULL,
0,
module_methods,
NULL,
NULL,
NULL,
NULL
};
PyMODINIT_FUNC
PyInit__xxtestfuzz(void)
{
PyObject *m = NULL;
if ((m = PyModule_Create(&_fuzzmodule)) == NULL) {
return NULL;
}
return m;
}